问题描述
在SwiftUI代码中,通过Core Data的NSManagedObjectContext获取Core Data数据时,发现存在如下提示:
Capture of 'context' with non-sendable type 'NSManagedObjectContext' in a '@Sendable' closure
提示行代码:
Task {
await fetchCryptoData(context: context)
lastUpdateDate = Date()
}
func fetchCryptoData(context: NSManagedObjectContext) async {
let task = URLSession.shared.dataTask(with: apiURL) { data, response, error in
for coin in decoded {
// 获取 Core Data 中的加密外币数据
let existingCryptos = try context.fetch(fetchRequest) // 提示行
let existingDict = Dictionary(uniqueKeysWithValues: existingCryptos.map{ ($0.id, $0) })
}
}
}
提示截图:
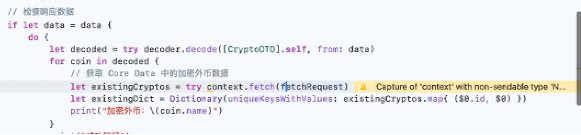
这个提示表示,在一个 @Sendable 闭包中捕获了一个非 Sendable 类型(这里是 NSManagedObjectContext),而 Swift Concurrency(并发)要求异步闭包(如 Task {} 或 URLSession.shared.data(for:))中的变量必须是 Sendable 的,以确保在多线程环境中不会造成数据竞争或崩溃。
问题原因
具体来讲,URLSession的回调闭包(escaping closure),它不会在主线程或 MainActor 上执行,也不会自动继承 Task {} 的上下文。
所以,这个闭包属于并发环境,不一定在主线程。
let task = URLSession.shared.dataTask(with: apiURL) { data, response, error in
// 这里是一个回调闭包
let existingCryptos = try context.fetch(fetchRequest)
}
而我在代码中使用async/await方法时,Swift 编译器会自动假设闭包是 @Sendable,也就是可以安全跨线程调用的闭包,并开始做类型追踪。如果捕获了 NSManagedObjectContext 这样的非 Sendable 类型,Swift 就会:
报出 “Capture of non-Sendable type in @Sendable closure” 的错误。
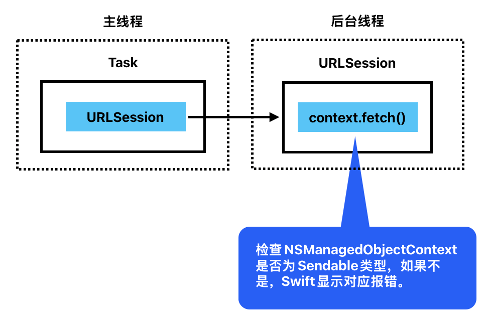
因为,如果在后台异步闭包中访问主线程的context,就会:
1)违反 Core Data 的线程隔离原则
2)触发 Swift Concurrency 检查警告或崩溃
解决方案
解决方案1: DispatchQueue.main.async
始终在主线程中调用context:
DispatchQueue.main.async {
// 主线程中使用 context,安全
context.fetch(...)
}
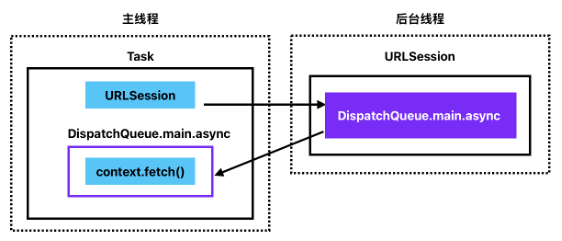
解决方案2: backgroundContext
或者使用Core Data后台专用的backgroundContext:
let backgroundContext = persistentContainer.newBackgroundContext()
backgroundContext.perform {
// 安全在线程隔离的 context 中 fetch/save
}
解决方案3: @MainActor
@MainActor
func fetchAndUpdate() async { ... }
这段代码会在主线程运行,不涉及跨线程数据传递,可以放心使用非Sendable的类型。
解决方案4:不使用async/await调用
因为我使用的是传统的 URLSession.dataTask 回调方式,因此完全可以不适应async/await来调用方法。
fetchCryptoData(context: context)
lastUpdateDate = Date()
func fetchCryptoData(context: NSManagedObjectContext) {
let task = URLSession.shared.dataTask(with: apiURL) { data, response, error in
for coin in decoded {
// 获取 Core Data 中的加密外币数据
let existingCryptos = try context.fetch(fetchRequest) // 提示行
let existingDict = Dictionary(uniqueKeysWithValues: existingCryptos.map{ ($0.id, $0) })
}
}
}
Swift 只会对 async let、Task {}、Task.detached {}、actor、@Sendable closure 等明确参与 Swift 并发模型的代码进行严格的类型检查。
当我不再使用async/await后,Swift 编译器不会将 context 的使用视为跨线程或并发访问,因此不会报错。
问题得到解决。
总结
在SwiftUI主线程中创建了NSManagedObjectContext,在方法中调用时,应该考虑不在后台线程、异步Task {}或URLSession的闭包中调用,本案例就是涉及在URLSession闭包中调用,导致Swift对NSManagedObjectContext进行严格的类型检查并报错。
因此,NSManagedObjectContext应该考虑在主线程中调用。
这个问题其实比较典型,特别是在并发和线程安全这块,因此应该理解主线程和其他线程直接可能存在的问题,以及解决方案。
扩展知识
1、Swift Sendable协议:https://fangjunyu.com/2025/04/07/swift-sendable%e5%8d%8f%e8%ae%ae/
2、Core Data NSManagedObjectContext的后台上下文backgroundContext:https://fangjunyu.com/2025/03/31/core-data-nsmanagedobjectcontext%e7%9a%84%e5%90%8e%e5%8f%b0%e4%b8%8a%e4%b8%8b%e6%96%87backgroundcontext/