在SwiftUI中,StrokeStyle 用于自定义线条的绘制方式,比如线宽、虚线、拐角形状等。可以在 .stroke(style:) 修饰符中传入一个 StrokeStyle 对象来自定义线条样式。
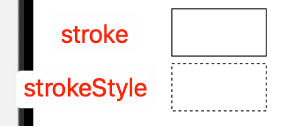
基本定义
struct StrokeStyle {
var lineWidth: CGFloat = 1
var lineCap: CGLineCap = .butt
var lineJoin: CGLineJoin = .miter
var miterLimit: CGFloat = 10
var dash: [CGFloat] = []
var dashPhase: CGFloat = 0
}
1、lineWidth:CGFloat类型,线条宽度。
2、lineCap:CGLineCap类型,线端形状(.butt / .round / .square)。
3、lineJoin:CGLineJoin类型,拐角连接样式(.miter / .round / .bevel)。
4、miterLimit:CGFloat类型,拐角尖锐度限制(对 .miter 有效)。
5、dash:[CGFloat]类型,虚线模式(如 [4,2] 表示4pt线 + 2pt空格循环)。
6、dashPhase:CGFload类型,虚线起始偏移量。
使用示例
1、普通实线:
Path { path in
path.move(to: CGPoint(x: 0, y: 0))
path.addLine(to: CGPoint(x: 100, y: 0))
}
.stroke(Color.blue, style: StrokeStyle(lineWidth: 2))
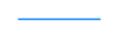
2、虚线:
Path { path in
path.addEllipse(in: CGRect(x: 0, y: 0, width: 100, height: 100))
}
.stroke(Color.green, style: StrokeStyle(lineWidth: 2, dash: [5, 3]))

[5, 3] 表示画 5pt,空 3pt,不断重复;
[10, 5, 2, 5] 表示画 10pt、空 5pt、画 2pt、空 5pt,循环。
3、改变线端与连接样式
Path { path in
path.move(to: CGPoint(x: 10, y: 10))
path.addLine(to: CGPoint(x: 60, y: 10))
path.addLine(to: CGPoint(x: 60, y: 60))
}
.stroke(Color.red, style: StrokeStyle(
lineWidth: 6,
lineCap: .round,
lineJoin: .round
))

lineCap 会影响线段末端的形状(圆头、平头、方头);
lineJoin 控制路径转角处的连接方式。
总结
使用StrokeStyle可以进一步丰富边框样式。
dash 值是长度数组,单位是点数(pt);
想要光滑虚线圆圈或曲线时,建议配合 .lineCap(.round);
在 SwiftUI Path 或 Shape 中配合 .stroke(style:) 使用。
相关文章
SwiftUI绘制边框stroke和strokeBorder:https://fangjunyu.com/2024/12/15/swiftui%e7%bb%98%e5%88%b6%e8%be%b9%e6%a1%86stroke%e5%92%8cstrokeborder/