在《汇率仓库》应用的开发过程中,想要实现一个汇率外币国旗和名称滚动的动画,例如下图中的底部两层国旗和名称向左滚动。
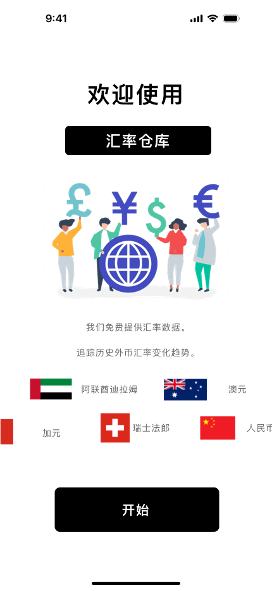
实现过程
首先把国旗的列表使用数组表示出来:
let countrys = [["AED","AUD","CAD","CHF","DKK","EUR","GBP","HKD","HUF","JPY","KRW","MOP"],["MXN","MYR","NOK","NZD","PLN","RUB","SAR","SEK","SGD","THB","TRY","USD","ZAR"]]
国旗列表数组内有两个不同的货币符号数组内容,用于区分上下两层动画。
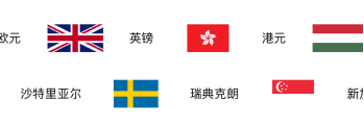
将国旗和货币名称列表,使用HStack实现出来:
HStack {
ForEach(countrys[item],id: \.self) { country in
Image("\(country)")
.resizable()
.scaledToFit()
.frame(width: 100)
Text(LocalizedStringKey(country))
.font(.footnote)
.fixedSize()
}
}
.frame(width: width,height: 30)
在这段代码中,Image需要设置固定的frame尺寸,Text需要设置fixedSize,否则HStack可能导致图片和文字被压缩的问题。
因为需要显示出两层国旗列表,我在外层再添加一个ForEach遍历:
ForEach(0..<2) { item in
HStack {
ForEach(countrys[item],id: \.self) { country in
Image("\(country)")
.resizable()
.scaledToFit()
.frame(width: 100)
Text(LocalizedStringKey(country))
.font(.footnote)
.fixedSize()
}
}
.frame(width: width,height: 30)
if item == 0 {
Spacer().frame(height: 30)
}
}
通过 ForEach(0..<2) 实现双层的效果。
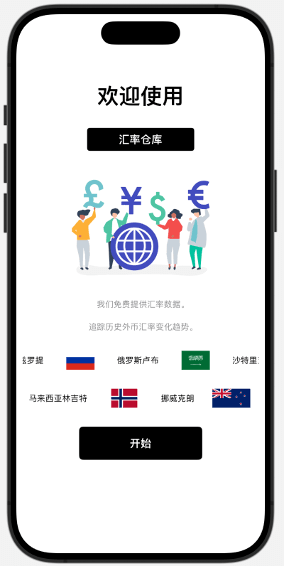
接下来是自动滚动动画的实现,这里主要依赖的是offset设置视图的偏移量,模仿实际的滚动效果。
HStack {
ForEach(countrys[item],id: \.self) { country in
Image("\(country)")
.resizable()
.scaledToFit()
.frame(width: 100)
Text(LocalizedStringKey(country))
.font(.footnote)
.fixedSize()
}
}
.offset(-100)
.frame(width: width,height: 30)
例如,我设置HStack的offset为x轴偏移-100,显示的实际效果就是向左偏移100的距离。
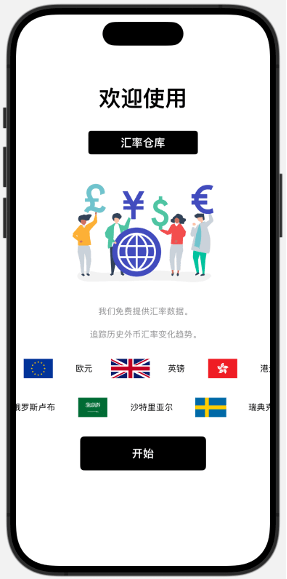
为了管理两层滚动视图的offset,需要先设置一个固定的offsets偏移变量。
@State private var offsets: [CGFloat] = [-730, -950]
这里需要根据HStack的宽度设置,例如我设置的偏移量分别为:-730和-950。
为什么设置-730和-950呢?是因为这两层HStack在设置为-730和-950以后,HStack基本都是偏移到末尾的状态,并且两个国旗列表之间会有一个偏差的间隙。
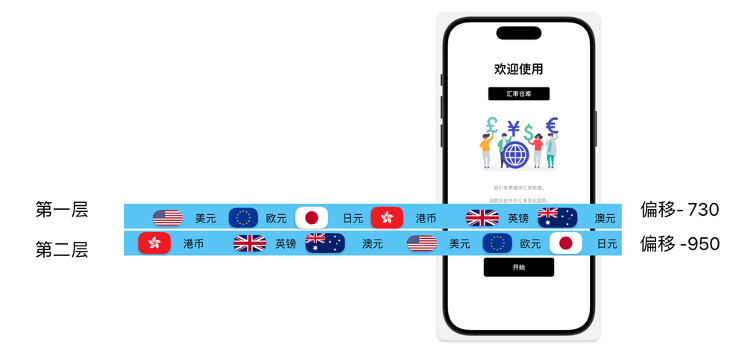
在实际应用中,具体的偏移量还需要自己根据视图位置进行设置,也可以使用geometryReader计算宽度并设置偏移量。
在ForEach遍历中,使用offsets[item]绑定对应的偏移变量。
ForEach(0..<2) { item in
HStack {
ForEach(countrys[item],id: \.self) { country in
Image("\(country)")
.resizable()
.scaledToFit()
.frame(width: 100)
Text(LocalizedStringKey(country))
.font(.footnote)
.fixedSize()
}
}
.offset(x:offsets[item])
.frame(width: width,height: 30)
if item == 0 {
Spacer().frame(height: 30)
}
}
设置offset绑定对应的offsets数组,这样就可以设置offsets数组中的每个值,使得绑定的视图进行偏移。
设置滚动动画
最后,新增一个 startAnimation 方法,通过withAnimation、Animation以及repeatForever属性实现平滑的动画效果。
在withAnimation中,将两个offsets数组值设置为0,这样,就会通过动画的效果,将实现两层国旗向左滚动的效果。
func startAnimation() {
withAnimation(Animation.linear(duration: animationDuration).repeatForever(autoreverses:false)) {
offsets[0] = 0
offsets[1] = 0
}
}
其中duration设置的animationDuration是一个@State变量,用于设置动画的持续时间:
let animationDuration: Double = 60
在国旗视图代码上,设置onAppear闭包调用startAnimation方法。
ForEach(0..<2) { item in
// 相关代码
}
.onAppear {
startAnimation()
}
运行Xcode预览,实现循环滚动的动画效果。
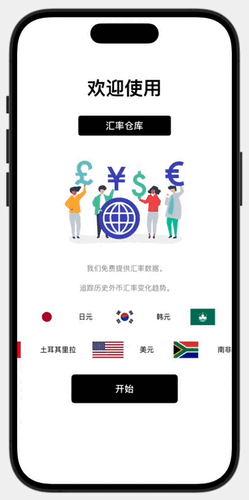
补充知识:无缝循环
动画基本实现,但是循环滚动的效果还不好,因为当我把持续时间设置为5秒时,可以看到动画在执行完毕后,会卡顿并返回到原来的起始位置并重新播放动画。
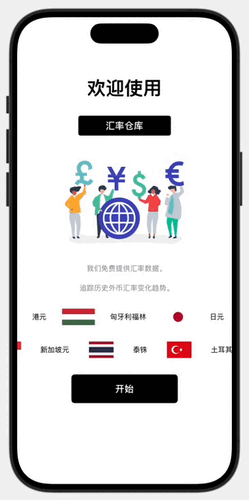
如果想要实现无缝循环动画,就需要使用关键偏移量重制。
无缝循环的原理:
1、滚动内容超过视图宽度时,重置 offset
动画持续滚动时,offset 不断向负方向移动。
当 offset 变化量等于内容总宽度的一半时,瞬间重置 offset 回到起点,形成无缝效果。
2、视图复制两份,但逻辑上只需要滚动一个宽度
在滚动完全结束时瞬间回到初始位置。
使用Timer进行手动更新
使用Timer进行手动更新后,不用使用 withAnimation(Animation.linear().repeatForever()),而是使用 Timer 每隔一段时间更新 offsets。
首先声明一个timer变量:
@State private var timer: Timer?
将原来的startAnimation方法修改为:
func startAnimation() {
let step: CGFloat = 3 // 每帧减少 3 像素
let interval = 0.01 // 计算时间间隔
timer = Timer.scheduledTimer(withTimeInterval: interval, repeats: true) { _ in
for i in 0..<2 {
withAnimation {
offsets[i] += step
}
// 当偏移量超过最大值时,重置为初始位置,实现无缝循环
if i == 0, offsets[i] >= 330 {
offsets[i] = -1550
} else if i == 1, offsets[i] >= 460 {
offsets[i] = -1800
}
}
}
}
startAnimation方法中的step设置每一次offset偏移的位置。Interval用于Timer的触发间隔,如果Interval为0.01,表示每0.01秒触发一次Timer代码。
在Timer中设置repeats为重复触发。
Timer每次触发,offsets都会递增step对应的偏移位置,比如上面代码中每0.01秒偏移3像素。
i==0表示第一个国旗滚动列表,当第一个列表的偏移大于330时,重置offset的位置。当第二个列表对应的offset大于460时,重置offset的位置。
这里的330和460是根据列表单独调整的,为了循环时跳转到对应的offset偏移设置的。
而-1550和1800也是我为了避免出现空白区域,再次调整的offsets偏移。
@State private var offsets: [CGFloat] = [-1550, -1800]
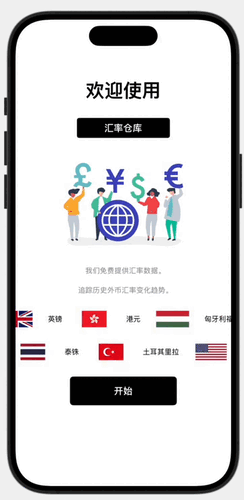
总结
实际上无缝循环的代码还是有一些问题,例如重置offset偏移时,还需要手动调整。如果想要实现非常流畅的无缝循环,应该还是需要GeometryReader计算整个HStack国旗列表的宽度,然后根据代码进行offset的偏移设置,而不是手动配置。
手动配置的结果一是不准,二是当应用需要本地化时,每种语言的间隔也不一样,因此这里的无缝循环只是作为一个引导,后续有更好的实现思路还是再补充。
以上就是全部的循环滚动动画的过程。
相关文章
1、HStack默认布局导致视图不显示的问题:https://fangjunyu.com/2025/03/31/hstack%e9%bb%98%e8%ae%a4%e5%b8%83%e5%b1%80%e5%af%bc%e8%87%b4%e8%a7%86%e5%9b%be%e4%b8%8d%e6%98%be%e7%a4%ba%e7%9a%84%e9%97%ae%e9%a2%98/
2、SwiftUI容器视图GeometryReader:https://fangjunyu.com/2024/12/15/swiftui%e5%ae%b9%e5%99%a8%e8%a7%86%e5%9b%begeometryreader/
3、SwiftUI动画animation:https://fangjunyu.com/2024/12/16/swiftui%e5%8a%a8%e7%94%bbanimation/
4、SwiftUI动画效果withAnimation:https://fangjunyu.com/2024/12/14/swiftui%e5%8a%a8%e7%94%bb%e6%95%88%e6%9e%9cwithanimation/
5、SwiftUI动画重复修饰符repeatForever:https://fangjunyu.com/2024/12/15/swiftui%e5%8a%a8%e7%94%bb%e9%87%8d%e5%a4%8d%e4%bf%ae%e9%a5%b0%e7%ac%a6repeatforever/
6、Swift和Foundation框架创建和管理定时任务的Timer类:https://fangjunyu.com/2024/12/14/swift%e5%92%8cfoundation%e6%a1%86%e6%9e%b6%e5%88%9b%e5%bb%ba%e5%92%8c%e7%ae%a1%e7%90%86%e5%ae%9a%e6%97%b6%e4%bb%bb%e5%8a%a1%e7%9a%84timer%e7%b1%bb/
附录
视图代码
import SwiftUI
struct WelcomeView: View {
@State private var offsets: [CGFloat] = [-1550, -1800]
let animationDuration: Double = 5
@Binding var ViewSteps: Int
@Environment(\.colorScheme) var color
let countrys = [
["AED","AUD","CAD","CHF","DKK","EUR","GBP","HKD","HUF","JPY","KRW","MOP"],
["MXN","MYR","NOK","NZD","PLN","RUB","SAR","SEK","SGD","THB","TRY","USD","ZAR"]
]
@State private var timer: Timer?
func startAnimation() {
let step: CGFloat = 1 // 每帧减少 3 像素
let interval = 0.01 // 计算时间间隔
timer = Timer.scheduledTimer(withTimeInterval: interval, repeats: true) { _ in
for i in 0..<2 {
withAnimation {
offsets[i] += step
}
// 当偏移量超过最大值时,重置为初始位置,实现无缝循环
if i == 0, offsets[i] >= 330 {
offsets[i] = -1550
} else if i == 1, offsets[i] >= 460 {
offsets[i] = -1800
}
}
}
}
var body: some View {
GeometryReader { geo in
let width = geo.frame(in: .global).width * 0.95
VStack {
Spacer().frame(height: 50)
// 设置最大宽度
VStack {
Text("Welcome")
.font(.largeTitle)
.fontWeight(.bold)
Spacer()
.frame(height: 30)
Text("ERdepot")
.fontWeight(.bold)
.padding(.vertical,8)
.padding(.horizontal,50)
.foregroundColor(color == .light ? .white : .black)
.background(color == .light ? .black : .white)
.cornerRadius(4)
Spacer().frame(height: 30)
Image("welcome")
.resizable()
.scaledToFit()
.frame(width: 240)
Spacer().frame(height: 30)
// 介绍文字
VStack {
Text("We provide free exchange rate data.")
Spacer().frame(height: 20)
Text("Track historical foreign exchange rate trends.")
}
.multilineTextAlignment(.center)
.foregroundColor(.gray)
.font(.footnote)
}
.frame(maxWidth: width)
Spacer().frame(height: 30)
ForEach(0..<2) { item in
HStack {
ForEach(0..<2) { _ in
ForEach(countrys[item],id: \.self) { country in
Image("\(country)")
.resizable()
.scaledToFit()
.frame(width: 100)
Text(LocalizedStringKey(country))
.font(.footnote)
.fixedSize()
}
}
}
.offset(x:offsets[item])
.frame(width: width,height: 30)
if item == 0 {
Spacer().frame(height: 30)
}
}
.onAppear {
startAnimation()
}
Spacer().frame(height: 30)
// 设置最大宽度
VStack {
Button(action: {
// 跳转到隐私视图
ViewSteps = 1
}, label: {
Text("Start")
.fontWeight(.bold)
.padding(.vertical,16)
.padding(.horizontal,80)
.foregroundColor(color == .light ? .white : .black)
.background(color == .light ? .black : .white)
.cornerRadius(6)
})
}
.frame(maxWidth: width)
Spacer()
}
.frame(maxWidth: .infinity,maxHeight: .infinity)
}
}
}
#Preview {
WelcomeView(ViewSteps: .constant(0))
}