在 SwiftUI 中,TextField 可以使用 FormatStyle 直接格式化用户输入的内容,格式化后的数据可以是数字、日期、时间、货币等。
基本语法
TextField("占位符", value: $变量, format: 格式)
value:与 @State 变量绑定的值
format:使用 FormatStyle 格式化数据
格式化数字输入
整数格式
import SwiftUI
struct ContentView: View {
@State private var number: Int? = nil
var body: some View {
VStack {
TextField("请输入数字", value: $number, format: .number)
.textFieldStyle(RoundedBorderTextFieldStyle())
.keyboardType(.numberPad)
.padding()
if let num = number {
Text("你输入的数字是:\(num)")
}
}
.padding()
}
}
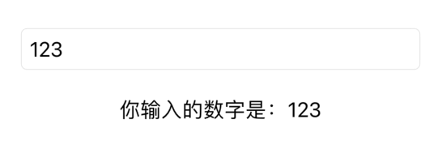
format: .number 会自动格式化为带有千分位的整数,例如:1,000。
自定义小数格式
@State private var value: Double = 0.0
TextField("输入小数", value: $value, format: .number.precision(.fractionLength(2)))
.textFieldStyle(RoundedBorderTextFieldStyle())
.keyboardType(.decimalPad)
该格式会保留两位小数,例如:12.34。
货币格式
@State private var price: Double = 0.0
TextField("输入金额", value: $price, format: .currency(code: "USD"))
.textFieldStyle(RoundedBorderTextFieldStyle())
.keyboardType(.decimalPad)
格式为货币,如:$1,234.56。
还可以根据需求更改货币符号,例如:”CNY”(人民币 ¥)或 “EUR”(欧元 €)。
百分比格式
@State private var percentage: Double = 0.5
TextField("输入百分比", value: $percentage, format: .percent)
.textFieldStyle(RoundedBorderTextFieldStyle())
.keyboardType(.decimalPad)
格式化成百分比,例如:50%。
格式化日期输入
基本日期格式
@State private var selectedDate: Date = Date()
TextField("选择日期", value: $selectedDate, format: .dateTime.year().month().day())
.textFieldStyle(RoundedBorderTextFieldStyle())
.padding()
格式为 2025-03-24。
自定义日期格式
@State private var selectedDate: Date = Date()
TextField("输入日期", value: $selectedDate, format: .dateTime.day().month().year())
.textFieldStyle(RoundedBorderTextFieldStyle())
格式为 24 Mar 2025,也可以根据 FormatStyle 来自定义格式。
时间格式
@State private var selectedTime: Date = Date()
TextField("输入时间", value: $selectedTime, format: .dateTime.hour().minute())
.textFieldStyle(RoundedBorderTextFieldStyle())
格式为 10:30 AM。
自定义 FormatStyle
如果 FormatStyle 无法满足需求,可以创建自己的格式化器。
创建一个自定义 FormatStyle
struct UppercaseFormatStyle: FormatStyle {
func format(_ value: String) -> String {
return value.uppercased()
}
}
在 TextField 中使用自定义 FormatStyle
@State private var text: String = ""
TextField("输入文本", value: $text, format: UppercaseFormatStyle())
.textFieldStyle(RoundedBorderTextFieldStyle())
这会将用户输入的文本转换为大写。
FormatStyle 适用的类型
TextField 的 format 参数支持的常见类型:
Int.FormatStyle – 整数
Double.FormatStyle – 小数
Date.FormatStyle – 日期/时间
Currency.FormatStyle – 货币
Percent.FormatStyle – 百分比
自定义 FormatStyle – 可根据需求创建自定义格式
总结
如果不使用FormatStyle,TextField默认只能绑定字符串格式。
TextField + FormatStyle 提供了灵活的数据格式化方案。
适用于整数、小数、货币、百分比、日期、时间等数据类型。
支持自定义 FormatStyle 以满足特殊需求。