在 SwiftUI 中,Section 是一种用于在 List 或 Form 中组织和分组内容的视图容器。它可以包含标题(header)、内容(content)以及页脚(footer),从而为数据提供逻辑上的分隔,并提升界面的可读性。
基本用法
import SwiftUI
struct SectionExampleView: View {
var body: some View {
List {
Section(header: Text("Fruits")) {
Text("Apple")
Text("Banana")
Text("Orange")
}
Section(header: Text("Vegetables"), footer: Text("Healthy choices!")) {
Text("Carrot")
Text("Broccoli")
Text("Spinach")
}
}
.listStyle(.insetGrouped) // 设置列表样式
}
}
struct SectionExampleView_Previews: PreviewProvider {
static var previews: some View {
SectionExampleView()
}
}
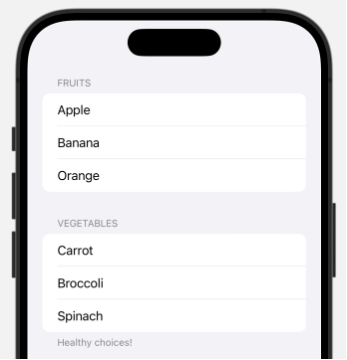
主要功能
1、Header(头部)
可以使用文字、图片或任何自定义视图作为标题。
Section(header: Text("Section Title")) {
Text("Item 1")
}
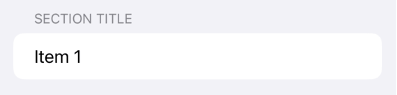
2、Footer(尾部)
用于在分组末尾添加说明性文字或其他元素。
Section(footer: Text("This is a footer")) {
Text("Item 1")
}
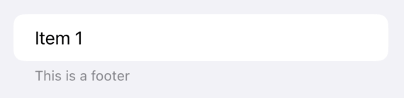
在实际场景中,可以实现在List底部添加说明文字。
3、自定义 Header 和 Footer
Section 支持任何视图作为 Header 或 Footer。
Section(header: HStack {
Image(systemName: "star")
Text("Custom Header")
}, footer: Text("Custom Footer")) {
Text("Item 1")
}
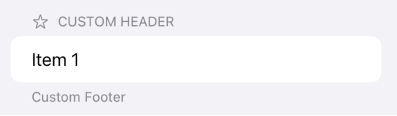
4、动态内容
可以使用 ForEach 动态生成内容。
let items = ["Apple", "Banana", "Orange"]
Section(header: Text("Fruits")) {
ForEach(items, id: \.self) { item in
Text(item)
}
}
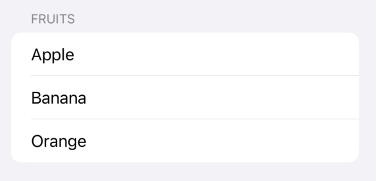
样式调整
List {
let items = ["Apple", "Banana", "Orange"]
ForEach(items, id: \.self) { item in
Text(item)
}
}
.listStyle(.sidebar) // 设置列表样式
可以通过 .listStyle 修改列表的显示样式:
1、.automatic(自动)
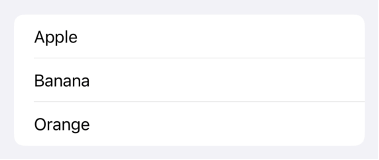
2、.grouped(分组)
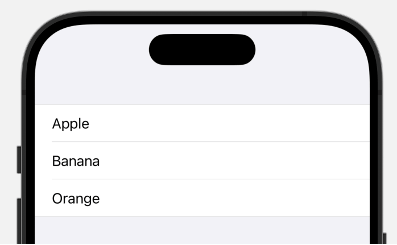
3、.inset(插入)
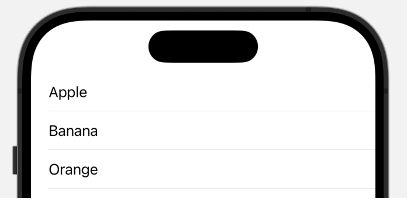
4、.insetGrouped(插入分组)
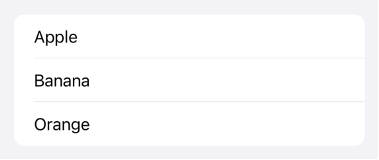
5、.plain(纯文本)
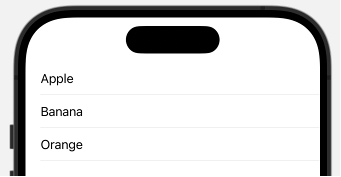
6、.sidebar(侧边栏)
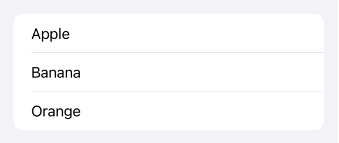
总结
Header 和 Footer:可以是文本或自定义视图。
动态内容:结合 ForEach 使用,支持大数据列表。
样式控制:通过 .listStyle 和 .listRowBackground 自定义外观。
嵌套与组合:灵活设计复杂的层次结构。
相关文章
SwiftUI在List底部显示注释或说明:https://fangjunyu.com/2025/01/13/swiftui%e5%9c%a8list%e5%ba%95%e9%83%a8%e6%98%be%e7%a4%ba%e6%b3%a8%e9%87%8a%e6%88%96%e8%af%b4%e6%98%8e/