SwiftUI 提供了许多视图组件,用于构建现代化的用户界面。这些组件功能丰富,支持响应式设计,并与 SwiftUI 的数据绑定系统无缝集成。以下是一些常用的 SwiftUI 视图组件分类及其示例:
布局组件
这些组件用于控制视图的布局和排列。
1、VStack
垂直排列子视图。
VStack {
Text("Hello")
Text("World")
}
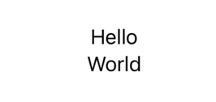
2、HStack
水平排列子视图。
HStack {
Image(systemName: "star")
Text("Favorite")
}
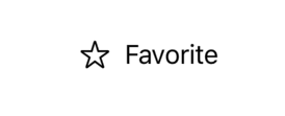
3、ZStack
将子视图叠加在一起。
ZStack {
Color.blue
Text("Hello")
.foregroundColor(.white)
}
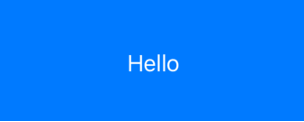
4、Grid(iOS 16+)
基于网格的布局。
Grid {
GridRow {
Text("A").background(Color.red)
Text("B").background(Color.blue)
}
GridRow {
Text("C").background(Color.green)
Text("D").background(Color.yellow)
}
}
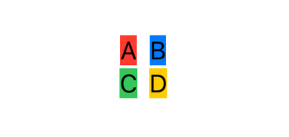
5、Spacer
在布局中添加弹性间距。
HStack {
Text("Left")
Spacer()
Text("Right")
}
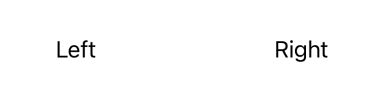
6、Divider
显示一条分割线。
VStack {
Text("Above Divider")
Divider()
Text("Below Divider")
}
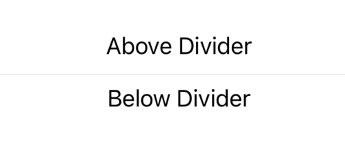
文本和图像
1、Text
显示静态或动态文本。
Text("Hello, SwiftUI!")
.font(.headline)
.foregroundColor(.blue)
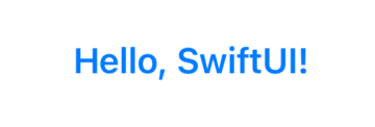
2、Image
显示本地或系统图标。
Image(systemName: "star.fill")
.resizable()
.frame(width: 50, height: 50)
.foregroundColor(.yellow)
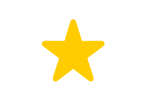
输入组件
1、Button
创建可交互的按钮。
Button("Tap Me") {
print("Button tapped!")
}
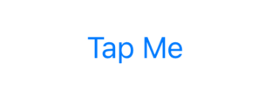
2、TextField
接受用户输入。
@State private var text = ""
TextField("Enter your name", text: $text)
.textFieldStyle(.roundedBorder)
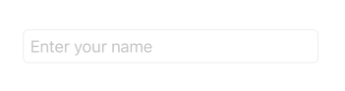
3、SecureField
接受密码输入。
@State private var password = ""
SecureField("Password", text: $password)
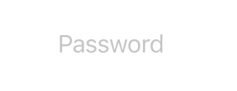
4、Toggle
开关组件。
@State private var isOn = false
Toggle("Enable Feature", isOn: $isOn)
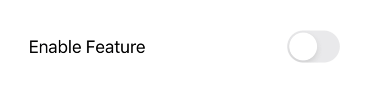
5、Slider
滑块组件。
@State private var value: Double = 0.5
Slider(value: $value, in: 0...1)
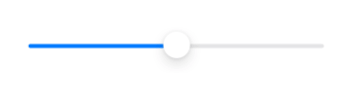
6、Picker
选择多个选项之一。
@State private var selectedOption = "Option 1"
let options = ["Option 1", "Option 2", "Option 3"]
Picker("Select an Option", selection: $selectedOption) {
ForEach(options, id: \.self) { option in
Text(option)
}
}
.pickerStyle(.wheel)
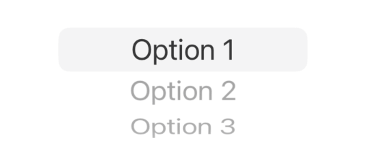
数据显示
1、List
显示垂直列表。
let items = ["Apple", "Banana", "Cherry"]
List(items, id: \.self) { item in
Text(item)
}
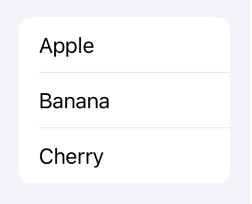
2、ScrollView
可滚动视图。
ScrollView {
VStack {
ForEach(1..<50) { index in
Text("Item \(index)")
}
}
}
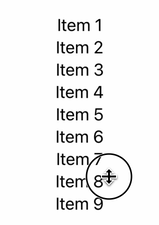
3、ForEach
遍历数据以生成视图。
ForEach(1..<6) { index in
Text("Item \(index)")
}
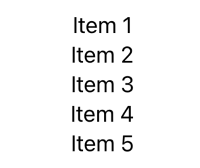
容器和导航
1、NavigationStack (iOS 16+)
支持导航功能。
NavigationStack {
NavigationLink("Go to Details", destination: Text("Details View"))
}
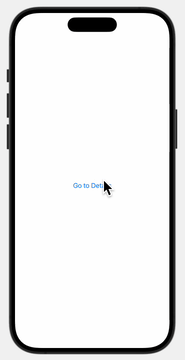
2、TabView
选项卡式视图。
TabView {
Text("Home")
.tabItem {
Label("Home", systemImage: "house")
}
Text("Profile")
.tabItem {
Label("Profile", systemImage: "person")
}
}
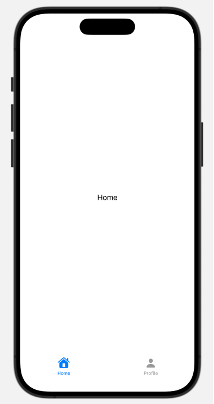
动画和修饰
1、ProgressView
显示进度指示器。
ProgressView("Loading...")
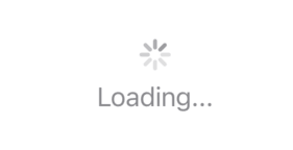
2、Alert
弹出警告对话框。
@State private var showAlert = false
Button("Show Alert") {
showAlert = true
}
.alert("Important Message", isPresented: $showAlert) {
Button("OK", role: .cancel) {}
}
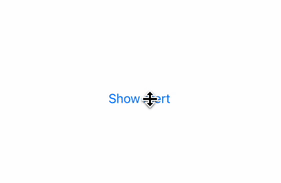
3、Sheet
以模态形式呈现视图。
@State private var showSheet = false
Button("Show Sheet") {
showSheet = true
}
.sheet(isPresented: $showSheet) {
Text("This is a sheet.")
}
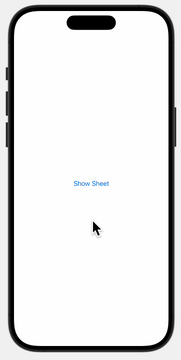
4、Animation
应用动画效果。
@State private var isAnimated = false
Rectangle()
.frame(width: isAnimated ? 200 : 100, height: 100)
.animation(.easeInOut, value: isAnimated)
.onTapGesture {
isAnimated.toggle()
}
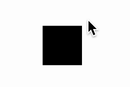
其他
1、Map (iOS 14+)
显示地图。
import MapKit
struct ContentView: View {
@State private var region = MKCoordinateRegion(
center: CLLocationCoordinate2D(latitude: 35.557267, longitude: 119.631039),
span: MKCoordinateSpan(latitudeDelta: 0.05, longitudeDelta: 0.05)
)
var body: some View {
Map(coordinateRegion: $region)
.edgesIgnoringSafeArea(.all)
}
}
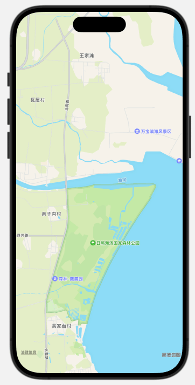
2、VideoPlayer (iOS 14+)
播放视频。
import AVKit
struct ContentView: View {
let player = AVPlayer(url: URL(string: " https://test-videos.co.uk/vids/bigbuckbunny/mp4/h264/720/Big_Buck_Bunny_720_10s_1MB.mp4")!)
var body: some View {
VideoPlayer(player: player)
.frame(height: 200)
}
}
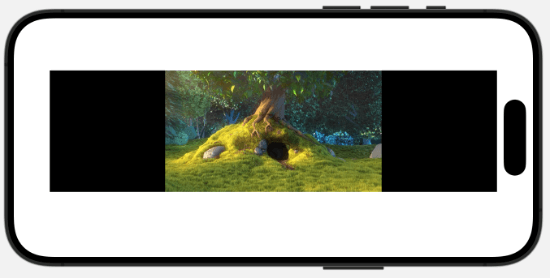