在 SwiftUI 中,ProgressView 是一个内置的进度指示器,可以显示任务的进度或简单的加载指示器。以下是其常见用法和自定义技巧:
基本用法
import SwiftUI
struct ContentView: View {
var body: some View {
ProgressView("Loading...")
.padding()
}
}
显示加载文本:ProgressView(“Loading…”) 会显示一个活动指示器和文本。
样式:默认使用系统样式的小圆形活动指示器。
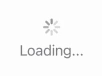
指定进度
可以显示一个明确的进度值(0.0 到 1.0):
import SwiftUI
struct ContentView: View {
@State private var progress: Double = 0.3
var body: some View {
VStack {
ProgressView(value: progress, total: 1.0)
.progressViewStyle(LinearProgressViewStyle())
.padding()
Button("Increase Progress") {
if progress < 1.0 {
progress += 0.1
}
}
}
}
}
value 和 total:分别表示当前进度值和最大值。
样式:LinearProgressViewStyle() 会显示线性进度条,而不是圆形活动指示器。
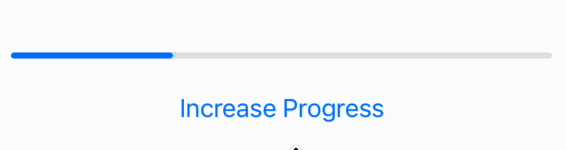
进度条样式
内置样式
SwiftUI 提供了两种样式:
1、CircularProgressViewStyle:圆形进度指示器。
ProgressView()
.progressViewStyle(CircularProgressViewStyle())
2、LinearProgressViewStyle:线性进度条。
ProgressView(value: 0.5)
.progressViewStyle(LinearProgressViewStyle())
自定义样式
可以自定义进度条样式:
struct CustomProgressViewStyle: ProgressViewStyle {
func makeBody(configuration: Configuration) -> some View {
ZStack {
RoundedRectangle(cornerRadius: 10)
.fill(Color.gray.opacity(0.3))
.frame(width: 200, height: 10)
RoundedRectangle(cornerRadius: 10)
.fill(Color.blue)
.frame(width: CGFloat(configuration.fractionCompleted ?? 0) * 200, height: 10)
}
}
}
struct ContentView: View {
@State private var progress: Double = 0.4
var body: some View {
ProgressView(value: progress)
.progressViewStyle(CustomProgressViewStyle())
.padding()
Button("Increase Progress") {
if progress < 1.0 {
progress += 0.1
}
}
}
}
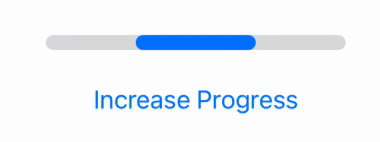
结合异步任务
ProgressView 可用于指示异步任务的加载状态:
struct ContentView: View {
@State private var isLoading = true
var body: some View {
VStack {
if isLoading {
ProgressView("Loading...")
} else {
Text("Task Complete!")
}
}
.onAppear {
// 模拟异步任务
DispatchQueue.main.asyncAfter(deadline: .now() + 2) {
isLoading = false
}
}
}
}
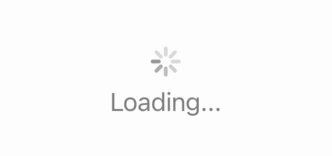
动画进度条
创建动态进度效果:
struct AnimatedProgressView: View {
@State private var progress: Double = 0.0
var body: some View {
ProgressView(value: progress, total: 1.0)
.progressViewStyle(LinearProgressViewStyle())
.padding()
.onAppear {
Timer.scheduledTimer(withTimeInterval: 0.1, repeats: true) { timer in
progress += 0.05
if progress >= 1.0 {
timer.invalidate()
}
}
}
}
}
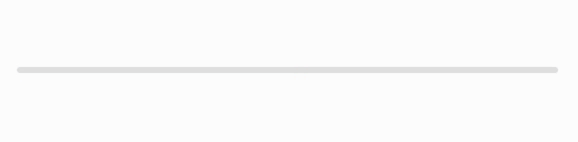
总结
ProgressView 在 SwiftUI 中功能灵活,适用于多种场景(任务指示、加载动画等)。可以通过样式、动画以及自定义样式来调整其外观,并结合异步任务使用。