scrollTargetLayout(isEnabled:) 是 SwiftUI 中的一种修饰符,用于启用或禁用子视图的滚动目标布局。默认情况下,isEnabled 参数设置为 true。
函数定义
public func scrollTargetLayout(isEnabled: Bool = true) -> some View
参数
isEnabled: 一个布尔值,用于指示滚动目标布局是否启用。
true:启用滚动目标布局。
false:禁用滚动目标布局。
作用
scrollTargetLayout 提供滚动视图中的布局支持,使视图能够在滚动过程中使用目标布局进行对齐或动画。它通常用于增强滚动行为,与 ScrollViewReader 或其他动态布局配合使用。
示例用法
1、启用滚动目标布局
struct ContentView: View {
var body: some View {
ScrollView {
LazyVStack {
ForEach(0..<10, id: \.self) { index in
Text("Item \(index)")
.frame(height: 100)
.frame(maxWidth: .infinity)
.background(Color.blue.opacity(0.3))
.scrollTargetLayout(isEnabled: true) // 启用布局
}
}
}
}
}
效果:
滚动视图会为每个子视图启用滚动目标布局。
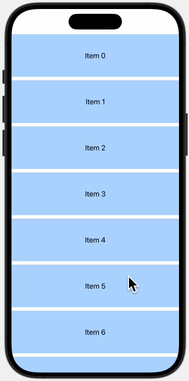
2、禁用滚动目标布局
struct ContentView: View {
var body: some View {
ScrollView {
LazyHStack {
ForEach(0..<10, id: \.self) { index in
Text("Item \(index)")
.frame(width: 100, height: 100)
.background(Color.orange.opacity(0.5))
.scrollTargetLayout(isEnabled: false) // 禁用布局
}
}
}
}
}
效果:
禁用滚动目标布局后,子视图不会在滚动目标计算中被使用。
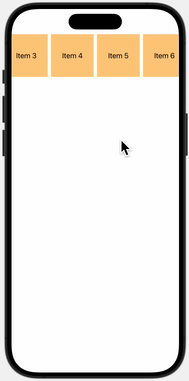
3、配合 ScrollViewReader 使用
struct ContentView: View {
var body: some View {
ScrollViewReader { proxy in
ScrollView {
LazyVStack {
ForEach(0..<50, id: \.self) { index in
Text("Item \(index)")
.frame(height: 80)
.frame(maxWidth: .infinity)
.background(Color.green.opacity(0.3))
.scrollTargetLayout(isEnabled: true) // 启用目标布局
.id(index) // 设置唯一标识符
}
}
}
.onAppear {
DispatchQueue.main.asyncAfter(deadline: .now() + 2) {
withAnimation {
proxy.scrollTo(25, anchor: .center) // 滚动到第 25 项
}
}
}
}
}
}
效果:
滚动视图可以精确滚动到目标子视图,并使用子视图的布局信息。
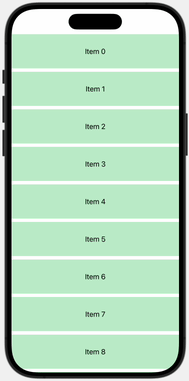
总结
1、功能简洁:scrollTargetLayout(isEnabled:) 用于控制子视图是否参与滚动目标布局的计算。
2、默认值:默认启用(isEnabled: true)。
3、灵活控制:通过布尔值参数动态启用或禁用布局。
4、典型场景:与 ScrollViewReader 结合使用,提供更细粒度的滚动行为控制。