在 SwiftUI 中,rotation3DEffect 是一个用于创建 3D 旋转效果的修饰符。它可以让视图在 3D 空间中围绕指定轴旋转。通过这个修饰符,可以实现类似立体翻转的动画效果。
基本语法
.rotation3DEffect(Angle, axis: (x: CGFloat, y: CGFloat, z: CGFloat), anchor: UnitPoint = .center, anchorZ: CGFloat = 0.0, perspective: CGFloat = 1.0)
参数说明
Angle: 旋转的角度,使用 Angle 类型,例如 .degrees(45)。
axis: 旋转轴的方向,由 x, y, z 三个分量组成。例如 (x: 1, y: 0, z: 0) 表示绕 X 轴旋转。
anchor: 旋转的锚点,默认为视图的中心点(UnitPoint.center)。
anchorZ: 控制锚点在 Z 轴上的位置,默认值为 0。
perspective: 控制透视投影的深度,默认值为 1。值越大,3D 效果越明显。
示例:
.rotation3DEffect(
.degrees(angle),
axis: (x: 1, y: 0, z: 0) // 绕 X 轴旋转
)
这段代码表示会根据angle的角度,围绕X轴进行旋转。
示例代码
基本旋转示例
struct Rotation3DEffectExample: View {
struct ContentView: View {
@State private var angle: Double = 0
var body: some View {
VStack {
Text("3D Rotation")
.font(.largeTitle)
.padding()
.rotation3DEffect(
.degrees(angle),
axis: (x: 1, y: 0, z: 0) // 绕 X 轴旋转
)
.animation(.easeInOut(duration: 1), value: angle)
Button("Rotate") {
angle += 180 // 增加旋转角度
}
}
}
}
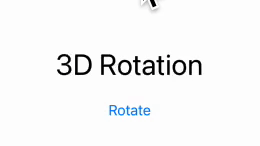
通过点击Button,可以调整Text文本旋转180度。
结合透视的旋转
struct PerspectiveRotationExample: View {
@State private var angle: Double = 0
var body: some View {
VStack {
Text("Perspective Rotation")
.font(.largeTitle)
.padding()
.rotation3DEffect(
.degrees(angle),
axis: (x: 0, y: 1, z: 0), // 绕 Y 轴旋转
perspective: 0.5 // 设置透视效果
)
.animation(.easeInOut(duration: 1), value: angle)
Button("Rotate") {
angle += 90
}
}
}
}
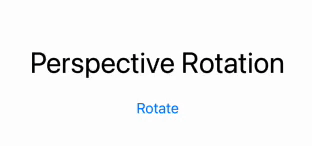
点击Button按钮,Text文本会按照Y轴宣传,这里添加了透视效果。
透视(Perspective)是一种视觉效果,用来模拟人眼观察到的三维世界中物体的深度和远近感。简单来说,它让你在屏幕上看到的物体看起来更像是在真实的 3D 空间中,而不是平面的。
在 SwiftUI 的 rotation3DEffect 中,perspective 参数决定了视图在进行 3D 旋转时的透视强度,即远近效果的真实感如何呈现。
perspective 参数的作用
默认值为 1.0,表示有一个适中的透视效果。
值越小(例如 0.5),透视效果越强烈,3D 旋转会显得更加立体,远近感更明显。
值越大(例如 2.0),透视效果减弱,接近于平面效果。
动态控制多个轴旋转
struct DynamicRotationExample: View {
@State private var xRotation: Double = 0
@State private var yRotation: Double = 0
@State private var zRotation: Double = 0
var body: some View {
VStack {
Text("Dynamic Rotation")
.font(.title)
.padding()
.rotation3DEffect(
.degrees(xRotation),
axis: (x: 1, y: 0, z: 0)
)
.rotation3DEffect(
.degrees(yRotation),
axis: (x: 0, y: 1, z: 0)
)
.rotation3DEffect(
.degrees(zRotation),
axis: (x: 0, y: 0, z: 1)
)
.animation(.spring(), value: xRotation)
.animation(.spring(), value: yRotation)
.animation(.spring(), value: zRotation)
VStack {
Slider(value: $xRotation, in: 0...360, label: { Text("X Rotation") })
Slider(value: $yRotation, in: 0...360, label: { Text("Y Rotation") })
Slider(value: $zRotation, in: 0...360, label: { Text("Z Rotation") })
}
.padding()
}
}
}
通过三个Slider来操作Text所绑定的三个rotation3DEffect修饰符。
分别控制X、Y和Z轴的旋转。
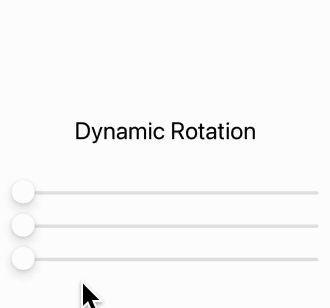
使用场景
1、卡片翻转效果:实现类似卡片翻面的 UI 动画。
2、动态视图旋转:在 3D 空间中显示旋转的 UI 元素。
3、增强视觉交互:配合 perspective 参数增加真实感。