在 SwiftUI 中,@Environment(\.accessibilityDifferentiateWithoutColor) 是一个非常有用的辅助功能环境值。它让应用知道用户是否启用了“无颜色区分”模式,这样可以动态调整 UI,以确保不依赖颜色传递信息。
什么是 accessibilityDifferentiateWithoutColor?
当用户在设备的 设置 > 辅助功能 > 显示与文字大小 > 不以颜色区分 中启用这个选项时,accessibilityDifferentiateWithoutColor 将被设置为 true。
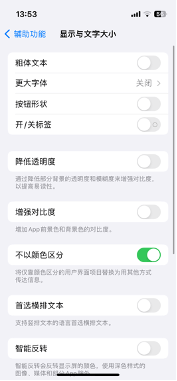
此时,应用应提供额外的非颜色区分的信息(例如,文本说明、图标或形状),以满足用户需求。
如何使用它?
以下是如何通过检测 accessibilityDifferentiateWithoutColor 来动态调整 UI 的示例:
示例 1:动态添加图标或文本
import SwiftUI
struct ContentView: View {
@Environment(\.accessibilityDifferentiateWithoutColor) var differentiateWithoutColor
var status: String = "success"
var body: some View {
HStack {
if differentiateWithoutColor {
// 提供文字或图标辅助信息
Image(systemName: status == "success" ? "checkmark.circle" : "xmark.circle")
.foregroundColor(status == "success" ? .green : .red)
} else {
// 仅使用颜色区分
Circle()
.fill(status == "success" ? .green : .red)
.frame(width: 20, height: 20)
}
Text(status == "success" ? "完成" : "错误")
}
.padding()
}
}
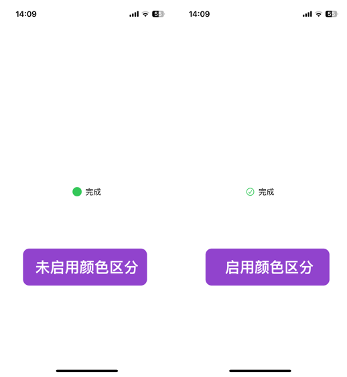
在这个例子中,如果用户启用了“无颜色区分”模式,应用会额外显示图标,而不仅仅通过颜色来区分状态。
示例 2:为按钮状态添加额外的形状提示
struct ContentView: View {
@Environment(\.accessibilityDifferentiateWithoutColor) var differentiateWithoutColor
var status: String = "active"
var body: some View {
HStack {
RoundedRectangle(cornerRadius: 10)
.fill(status == "active" ? Color.blue : Color.gray)
.frame(width: 100, height: 50)
.overlay(
Group {
if differentiateWithoutColor {
Text(status == "active" ? "启用" : "禁用")
.foregroundColor(.white)
}
}
)
.overlay(
Group {
if differentiateWithoutColor {
RoundedRectangle(cornerRadius: 10)
.stroke(Color.white, lineWidth: 2)
}
}
)
}
}
}
在这里,如果“无颜色区分”被启用,按钮还会显示文字或边框提示。
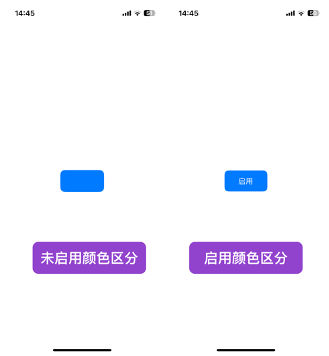
示例 3:游戏应用中的视觉提示
假设开发了一款需要用颜色区分状态的游戏(例如记忆游戏),在“无颜色区分”模式下,可以添加额外的图案或动画提示:
struct GameView: View {
@Environment(\.accessibilityDifferentiateWithoutColor) var differentiateWithoutColor
var isMatched: Bool = false
var body: some View {
ZStack {
if isMatched {
Circle()
.fill(Color.green)
if differentiateWithoutColor {
Text("✔")
.font(.largeTitle)
.foregroundColor(.white)
}
} else {
Circle()
.fill(Color.red)
if differentiateWithoutColor {
Text("✖")
.font(.largeTitle)
.foregroundColor(.white)
}
}
}
.frame(width: 100, height: 100)
}
}
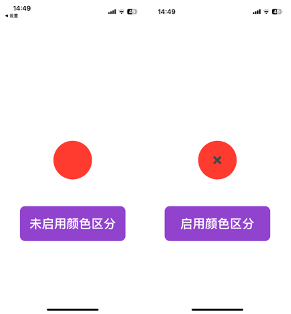
测试方式
可以在模拟器或设备上进行以下测试:
1、启用无颜色区分模式:
打开设备设置。
导航到 辅助功能 > 显示与文字大小 > 不以颜色区分,启用此选项。
2、运行应用并验证 accessibilityDifferentiateWithoutColor 的影响。
总结
属性来源:@Environment(\.accessibilityDifferentiateWithoutColor) 检测用户是否启用无颜色区分模式。
动态适配:根据该值调整应用的 UI,使其更具包容性。
结合其他特性:与 VoiceOver、accessibilityLabel 一起使用,提供更全面的辅助体验。
这种实现方式不仅提升了应用的用户体验,还展示了对不同用户群体的关怀。
参考文章
Supporting specific accessibility needs with SwiftUI:https://www.hackingwithswift.com/books/ios-swiftui/supporting-specific-accessibility-needs-with-swiftui