在 iOS 应用中,当需要在 background 阶段 执行长时间任务(如后台下载、音频播放或位置更新)时,必须在 Info.plist 文件中配置后台权限。这是因为 iOS 系统会对后台任务进行严格限制,未声明权限的任务可能会被中断。
如何配置 Info.plist
1、添加 Required background modes键
键名:Required background modes
类型:数组(Array)
描述:声明应用支持的后台模式,每种模式对应一个字符串值。
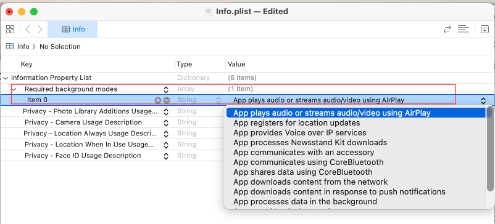
2、常见后台模式的值
以下是 Required background modes支持的常见值,以及对应的任务场景:
1、App plays audio or streams audio/video using AirPlay:应用需要在后台播放音频或视频内容,或通过 AirPlay 流媒体传输。
应用场景:音乐播放器、视频流媒体播放应用(如 Spotify、YouTube)。
2、App registers for location updates:应用需要持续获取地理位置信息,即使在后台。
应用场景:导航应用(如 Google Maps)、跑步跟踪器(如 Nike Run Club)。
3、App provides Voice over IP services:应用提供 VoIP(网络电话)服务,需持续监听传入呼叫。
应用场景:VoIP 服务(如 Skype、WhatsApp)。
4、App processes Newsstand Kit downloads:应用处理 iOS Newsstand(现已废弃)的背景下载。
应用场景:(已过时,适用于旧版 iOS)。
5、App communicates with an accessory:应用与外部设备配件进行通信(如使用特定硬件接口)。
应用场景:需要专用硬件的应用(如医疗设备、智能家居控制设备)。
6、App communicates using CoreBluetooth:应用作为蓝牙中心角色(Bluetooth Central),与蓝牙外设通信。
应用场景:蓝牙设备管理应用(如健康设备连接)。
7、App shares data using CoreBluetooth:应用作为蓝牙外围设备(Bluetooth Peripheral),广播数据。
应用场景:蓝牙传感器或信标广播设备。
8、App downloads content from the network:应用需要从网络下载内容(长时间运行下载任务)。
应用场景:文件下载器(如云存储应用)、大型资源加载器(如游戏)。
9、App downloads content in response to push notifications:应用在收到推送通知后下载内容。
应用场景:消息或新闻应用(如邮件客户端、新闻聚合器)。
10、App processes data in the background:应用在后台处理数据(例如复杂的计算任务)。
应用场景:数据处理或分析应用(如 AI 模型训练、数据清洗工具)。
11、App uses Nearby Interaction:应用使用 Nearby Interaction API 与附近的设备交互。
应用场景:依赖超宽带 (UWB) 的近场设备应用。
12、App uses Push-to-Talk:应用支持 “一键对讲” 功能,允许实时语音传输。
应用场景:对讲机类应用(如对讲功能软件)。
实现具体的后台任务
1、后台下载
使用 URLSession 的 background 配置,结合后台模式 fetch:
let config = URLSessionConfiguration.background(withIdentifier: "com.example.app.backgroundDownload")
let session = URLSession(configuration: config)
let task = session.downloadTask(with: URL(string: "https://example.com/largefile.zip")!)
task.resume()
background(withIdentifier:):创建一个支持后台运行的 URLSession。
后台处理下载结果:需要在 AppDelegate 的 application(_:handleEventsForBackgroundURLSession:completionHandler:) 中实现回调。
2、音频播放
使用 AVAudioSession 配置音频后台播放模式,并声明 audio 模式:
import AVFoundation
func setupAudioSession() {
do {
let audioSession = AVAudioSession.sharedInstance()
try audioSession.setCategory(.playback, mode: .default)
try audioSession.setActive(true)
} catch {
print("Failed to set up audio session: \(error)")
}
}
3、定期后台数据拉取
使用 application(_:performFetchWithCompletionHandler:) 方法处理后台刷新,结合 fetch 模式:
func application(_ application: UIApplication, performFetchWithCompletionHandler completionHandler: @escaping (UIBackgroundFetchResult) -> Void) {
fetchData { success in
completionHandler(success ? .newData : .noData)
}
}
注意事项
1、声明后台权限:
未在 Info.plist 声明的模式无法正常运行。
未使用或滥用后台模式可能导致 App Store 审核拒绝。
2、电池消耗与性能:
后台任务会增加电池消耗,应尽量减少后台任务的运行时间。
应用程序应在后台完成任务后尽快停止(如使用 endBackgroundTask)。
3、时间限制:
除 audio 和 location 外,大部分后台任务有严格的时间限制(通常为几分钟)。
4、用户隐私:
使用 location 时,必须在 Info.plist 中添加地理位置隐私声明(如 NSLocationWhenInUseUsageDescription)。
5、兼容性:
某些后台模式如 processing 仅在 iOS 13 或更高版本支持。
6、审查风险:
如果声明的后台模式与实际功能不匹配,审核时会被拒。
不应随意声明非必要的后台模式。
总结
在后台执行任务时:
必须在 Info.plist 中通过 UIBackgroundModes 声明所需权限。
配合具体的 API(如 URLSession、AVAudioSession)正确实现任务逻辑。
注意资源消耗与隐私保护,确保应用在满足需求的同时获得用户和 App Store 的认可。