在 SwiftUI 中,offset 是一种修饰符,用于调整视图在其父容器中的 相对位置。
基本语法
.offset(x: CGFloat, y: CGFloat)
参数:
x:视图在水平方向的偏移量。
y:视图在垂直方向的偏移量。
正值表示向右/向下偏移,负值表示向左/向上偏移。
示例代码
简单示例
struct ContentView: View {
var body: some View {
Text("Hello, World!")
.background(Color.blue)
.offset(x: 50, y: 20) // 向右偏移 50,向下偏移 20
.background(Color.red) // 红色背景是原始位置
}
}
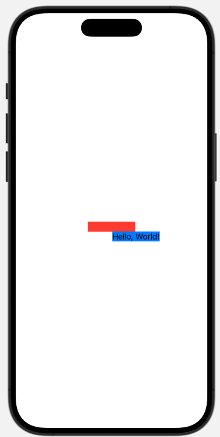
效果:
“Hello, World!” 的文字向右下方偏移 50x 和 20y。
蓝色背景跟随文字偏移。
红色背景显示的是视图原始位置。
动态偏移
结合状态变量,可以动态调整偏移量:
struct ContentView: View {
@State private var offsetAmount = CGSize.zero
var body: some View {
Text("Drag me!")
.padding()
.background(Color.green)
.offset(offsetAmount) // 偏移视图
.gesture(
DragGesture()
.onChanged { value in
offsetAmount = value.translation // 实时更新偏移量
}
.onEnded { _ in
offsetAmount = .zero // 手势结束后重置
}
)
}
}
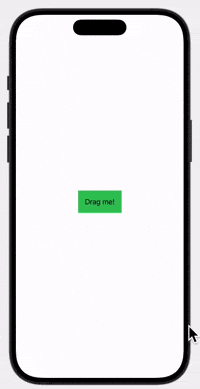
效果:
“Drag me!” 文本会跟随手指移动。
手势结束后,文字回到原位。
使用注意点
1、offset 仅调整视图的位置,不改变视图在父布局中的占位。
视图原本的位置仍占据空间。
用 position 可以真正改变视图的位置,放弃原始占位。
对比示例:
struct ContentView: View {
var body: some View {
VStack {
Text("Hello, World!") // 原始位置
.background(Color.blue)
.offset(x: 50) // 偏移但仍占空间
Rectangle()
.fill(Color.red)
.frame(height: 100)
}
}
}
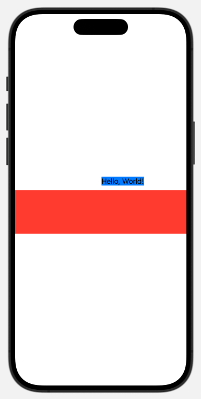
2、和 padding 配合使用:
如果视图有 padding,offset 的偏移量是基于视图的内容范围,而不是整个视图框架。
典型用途
1、实现动画效果
使用 withAnimation,轻松实现视图偏移动画:
struct ContentView: View {
@State private var moveRight = false
var body: some View {
Text("Slide me!")
.offset(x: moveRight ? 200 : 0) // 动态偏移量
.animation(.easeInOut, value: moveRight) // 添加动画
.onTapGesture {
moveRight.toggle()
}
}
}
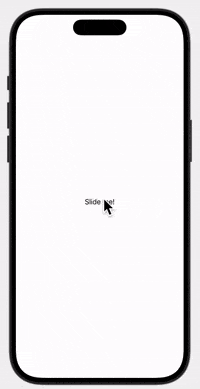
2、小范围调整视图布局
适合在视觉上微调某些视图的位置,不破坏整个布局。
3、与手势配合
拖拽手势、滑动动画等场景中,实时调整视图位置。
总结
offset:简单有效地调整视图的显示位置,不改变其占用的布局空间。
动态调整:结合手势和动画,可以实现复杂的交互效果。